Framer Motion: An In-Depth Guide to Animating Motion Values
Written on
Chapter 1: Introduction to Framer Motion
Framer Motion is a robust library that simplifies the process of rendering animations within React applications. This guide will provide a comprehensive overview of how to begin utilizing Framer Motion's capabilities, particularly focusing on the animate function.
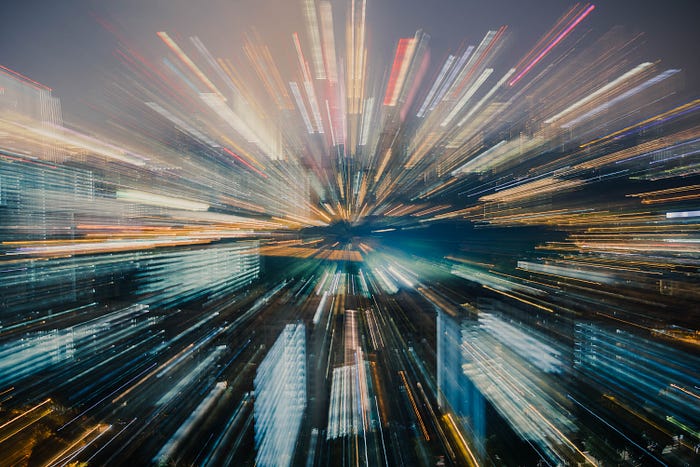
Section 1.1: Getting Started with Animation
To animate motion values, we can harness the power of the animate function. Here’s a simple example:
import React, { useEffect } from "react";
import { animate, motion, useMotionValue } from "framer-motion";
export default function App() {
const x = useMotionValue(0);
useEffect(() => {
const controls = animate(x, 100, {
type: "spring",
stiffness: 2000,
onComplete: (v) => {}
});
return controls.stop;
});
return (
<motion.div style={{ x }} />);
}
In this snippet, the useMotionValue hook creates an x motion value that can be animated. The animate function is called with parameters that define the animation type and its stiffness. We ensure to stop the animation when the component unmounts by returning the controls.stop method.
Section 1.2: Utilizing the Transform Function
The transform function allows us to map motion values to different outputs. Below is an example of how to convert a motion value into an opacity value:
import React, { useEffect, useState } from "react";
import { motion, transform, useMotionValue } from "framer-motion";
export default function App() {
const x = useMotionValue(0);
const inputRange = [0, 100];
const outputRange = [1, 0.3];
const [opacity, setOpacity] = useState();
useEffect(() => {
x.onChange((latest) => {
const opacity = transform(latest, inputRange, outputRange);
setOpacity(opacity);
});
}, []);
return (
<motion.div style={{ opacity }} />);
}
This code monitors changes in the x value using the onChange method. When the x value updates, it calculates the corresponding opacity using the transform function and adjusts the div's opacity accordingly.
Chapter 2: Advanced Transformations
In addition to simple transformations, we can create more complex mappings of motion values. Here’s a further example:
import React, { useEffect, useState } from "react";
import { motion, transform, useMotionValue } from "framer-motion";
export default function App() {
const x = useMotionValue(0);
const inputRange = [0, 100];
const outputRange = [1, 0.3];
const [opacity, setOpacity] = useState();
useEffect(() => {
x.onChange((latest) => {
const convertRange = transform(inputRange, outputRange);
const opacity = convertRange(x.get());
setOpacity(opacity);
});
}, []);
return (
<motion.div style={{ opacity }} />);
}
This example showcases how to use the convertRange function to dynamically adjust the opacity based on the latest x value.
The first video titled "Animation with Framer-Motion - #12 using motionValue" explores using motion values in various animation scenarios, providing practical insights into the Framer Motion library.
The second video, "Making a Realistic Folding Animation With Framer Motion," demonstrates how to create complex folding animations, emphasizing the library's versatility.
Conclusion
In conclusion, Framer Motion offers a powerful set of tools to animate motion values seamlessly. By utilizing the animate function and transform capabilities, developers can enhance the interactivity and dynamism of their React applications.