How to Adjust Anchor Links to Scroll Above Their Target with JavaScript
Written on
Chapter 1 Understanding Anchor Links
At times, we might want an anchor link to scroll slightly above its designated target. This guide will demonstrate how to achieve that using JavaScript.
Section 1.1 Utilizing the window.scrollTo Method
To scroll to specific coordinates on a webpage when a link with a hash in its href is clicked, we can leverage the window.scrollTo method.
For example, the following HTML snippet can be used:
<a href='#'>link 1</a>
<a href='#foo'>link 2</a>
<a href='#bar'>link 3</a>
<div id='bar' style='height: 500px'>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</div>
<div id='foo' style='height: 500px'>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</div>
Next, we define a function to handle the offset:
const offsetAnchor = () => {
if (location.hash.length !== 0) {
window.scrollTo(window.scrollX, window.scrollY - 100);}
};
We then select all anchor tags that contain a hash in their href attribute:
const anchors = document.querySelectorAll('a[href^="#"]');
for (const a of anchors) {
a.addEventListener('click', (event) => {
window.setTimeout(() => {
offsetAnchor();}, 0);
});
}
Additionally, we add an event listener to the document itself:
document.addEventListener('click', (event) => {
window.setTimeout(() => {
offsetAnchor();}, 0);
});
Finally, we invoke the offsetAnchor function initially to ensure that the page starts at the correct coordinates.
Subsection 1.1.1 Example Code
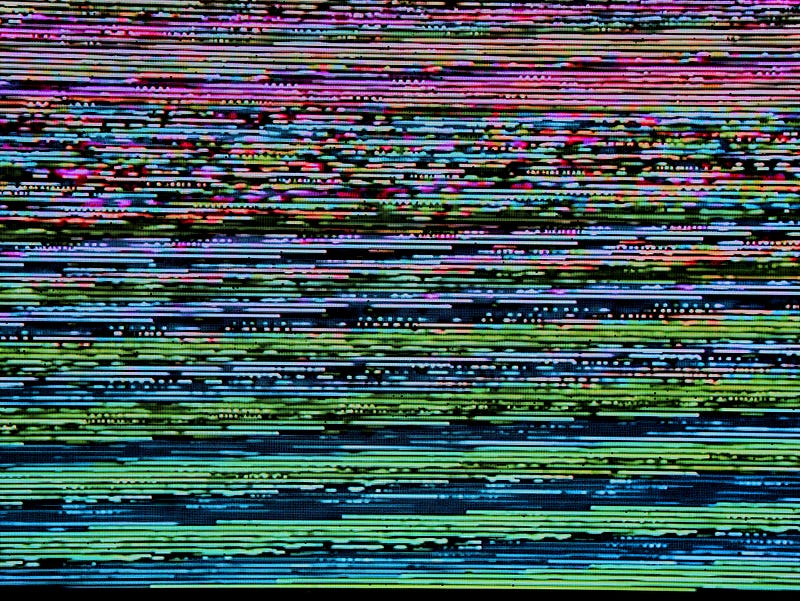
Section 1.2 Conclusion
In summary, the window.scrollTo method can be effectively used to adjust the scroll position when clicking on anchor links with hashes. This technique ensures a smoother user experience by allowing for slight adjustments in scroll position.
Chapter 2 Further Resources
For more insights, consider visiting PlainEnglish.io. You can also subscribe to our free weekly newsletter and follow us on various platforms like Twitter, LinkedIn, YouTube, and Discord. If you’re interested in Growth Hacking, check out Circuit for more information.