# Transforming Raw Data: Mastering Feature Engineering in Python
Written on
Chapter 1: Understanding Feature Engineering
Feature engineering plays a crucial role in converting unprocessed data into valuable features that can enhance the efficacy of machine learning algorithms. This article delves into the importance of feature engineering, supplemented with hands-on Python examples.
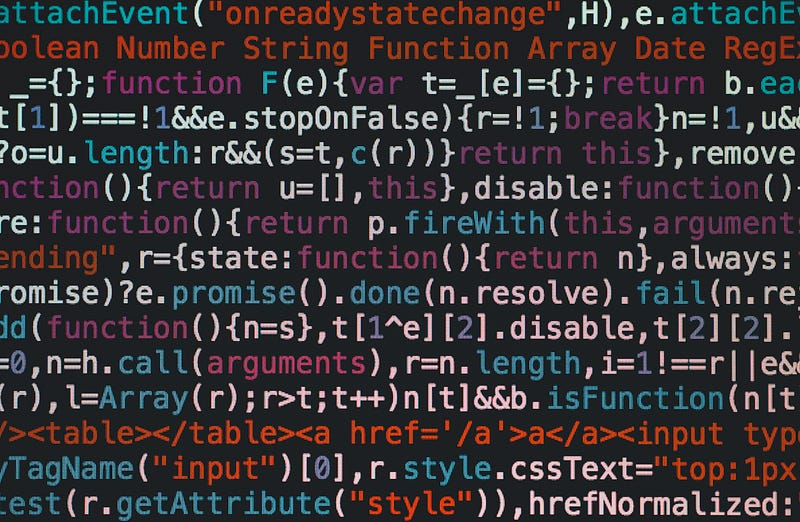
The Importance of Feature Engineering
In the realm of machine learning, data serves as the foundation, and the quality of features often surpasses the significance of the algorithm itself. Effective feature engineering can:
- Boost Model Accuracy: Skillfully constructed features can lead to better generalization, thus enhancing accuracy.
- Minimize Overfitting: Thoughtfully designed features help create models that are more resilient and less likely to overfit the training data.
- Improve Interpretability: Crafting insightful features allows for a deeper understanding of the model’s prediction mechanisms.
Let's explore several widely-used feature engineering methods through practical code examples.
1. Addressing Missing Data
Handling missing values effectively is essential. You can replace missing entries with a fixed value, the mean, median, or even develop a binary column to indicate the absence of data.
import pandas as pd
# Replace missing values with the mean
data['age'].fillna(data['age'].mean(), inplace=True)
# Generate a binary column for missing values
data['has_missing_age'] = data['age'].isnull().astype(int)
2. Encoding Categorical Variables
To process categorical data, it’s necessary to transform it into a numerical format. Techniques like one-hot encoding or label encoding can be employed.
# One-hot encoding
data = pd.get_dummies(data, columns=['gender', 'city'])
# Label encoding
from sklearn.preprocessing import LabelEncoder
label_encoder = LabelEncoder()
data['education'] = label_encoder.fit_transform(data['education'])
3. Creating Interaction Features
The relationship between two features can yield valuable insights.
# Generating an interaction feature
data['income_age_ratio'] = data['income'] / data['age']
4. Binning
Continuous variables can be categorized through binning.
# Categorizing ages
bins = [0, 18, 30, 50, 100]
labels = ['<18', '18-30', '30-50', '50+']
data['age_group'] = pd.cut(data['age'], bins=bins, labels=labels)
5. Feature Scaling
Scaling ensures that all features contribute equally to the model’s performance.
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
data['income_scaled'] = scaler.fit_transform(data[['income']])
6. Extracting Date-Time Features
Gleaning information from date-time variables can be beneficial.
# Extracting the month and day of the week
data['month'] = data['timestamp'].dt.month
data['day_of_week'] = data['timestamp'].dt.dayofweek
Conclusion
Feature engineering is a creative endeavor that necessitates a thorough understanding of your dataset and domain expertise. By leveraging these techniques, you can maximize your data's potential and develop more precise machine learning models.
Keep in mind that there isn’t a universal strategy for feature engineering. Experiment with various methods and let the characteristics of your data guide you in creating features that elevate your models.
Thank you for engaging with this content! Explore more insightful articles on my page!
Chapter 2: Video Insights on Feature Engineering
An introductory tutorial on feature engineering techniques in Python, perfect for beginners and advanced learners alike.
Discover various feature engineering techniques for machine learning in Python, enhancing your data science skillset.