Creating a Collapsible UITableViewCell in Swift: A Beginner's Guide
Written on
Introduction to Collapsible UITableViewCell
In this tutorial, we will explore the process of creating a collapsible UITableViewCell using Swift. This guide is suitable for beginners and utilizes Xcode 15.0 along with Swift 5.9.
Prerequisites
To follow along, you should have a basic understanding of Swift programming and UIKit.
Project Overview
I am developing a social media-style application that features a simple UITableView populated with UITableViewCell instances. Each time a cell is tapped, the like count will increase. The following implementation should be straightforward.
Data Management
Since there is no backend involved, we will create a simple data structure to manage the state of our cells.
struct CellData {
var likeCount: Int
var expanded: Bool
}
This structure will hold the likeCount as a variable, initialized to zero. In a full application, this value would typically be provided by a backend service. The expanded property will help manage the visibility of additional content in the cell.
Implementing Cell Expansion
When a user taps on a cell, it should expand to reveal more information. To achieve this, we'll manage the visibility of the relevant views based on the cell's state.
Here's how to create our custom UITableViewCell, named CollapsibleCell, which will handle the expansion feature.
final class CollapsibleCell: UITableViewCell {
private let counterLabel = UILabel()
private let button = UIButton()
private let stackView = UIStackView()
var onButtonClicked: (() -> Void)?
var count = 0 {
didSet {
counterLabel.text = "Like count: (count.description)"}
}
let expandableView: UIView = {
let view = UIView()
view.translatesAutoresizingMaskIntoConstraints = false
view.isHidden = true
return view
}()
// Initialization and setup...
}
The CollapsibleCell class includes a label to display the like count, a button for interaction, and a stack view to organize the layout.
Configuring the Table View
In our view controller, we will set up the table view and its data source. The cellForRowAt method will configure each cell based on the data model.
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
guard let cell = tableView.dequeueReusableCell(withIdentifier: Constants.cellReuseIdentifier) as? CollapsibleCell else {
debugPrint("Error: Unable to dequeue cell")
return UITableViewCell()
}
cell.selectionStyle = .none
let cellData = data[indexPath.row]
cell.expandableView.isHidden = !cellData.expanded
cell.count = cellData.likeCount
cell.onButtonClicked = { [weak self] in
self?.updateData(with: indexPath)}
return cell
}
Whenever a button in the cell is clicked, it triggers an update function that modifies the corresponding data and refreshes the specific cell.
Full Implementation Code
Below is the complete code for the collapsible table view cell controller.
final class CollapsibleTableCellViewController: UIViewController {
struct CellData {
var likeCount: Int
var expanded: Bool
}
// TableView and data setup...
}
This structure sets up the table view and its components, ensuring that everything works harmoniously.
Conclusion
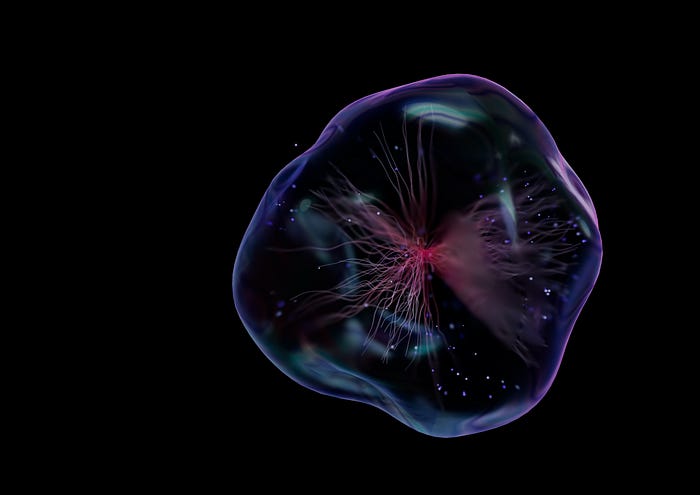